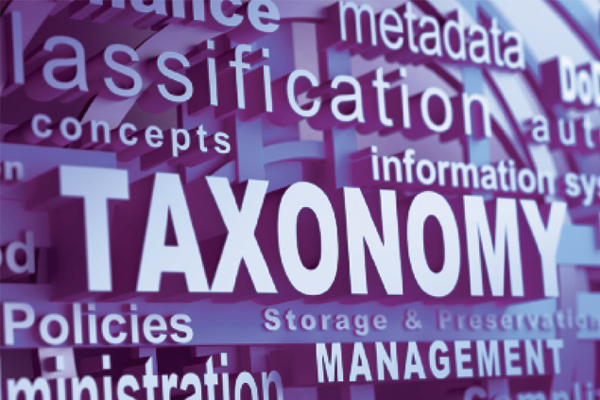
In Drupal, managing taxonomies plays a pivotal role in organizing and classifying content efficiently. Harnessing the power of programmatically creating vocabularies and terms empowers developers with dynamic control over taxonomy structures. Through programmatic manipulation, developers can seamlessly create, modify, and associate taxonomies with content types, providing a flexible and scalable approach to categorizing content within the Drupal ecosystem. Let's explore the methods to programmatically craft vocabularies and terms, enabling fine-grained control over taxonomy management in Drupal's content architecture.
1. Creating a Vocabulary:
To create a vocabulary programmatically, you'll use the \Drupal\taxonomy\Entity\Vocabulary class:
use Drupal\taxonomy\Entity\Vocabulary;
// Create a new vocabulary.
$vocabulary = Vocabulary::create([
'vid' => 'custom_vocabulary',
'name' => 'Custom Vocabulary',
'description' => 'This is a custom vocabulary.',
]);
$vocabulary->save();
2. Adding Terms to a Vocabulary:
Adding terms programmatically involves using the \Drupal\taxonomy\Entity\Term class:
use Drupal\taxonomy\Entity\Term;
// Load the vocabulary.
$vocabulary = \Drupal\taxonomy\Entity\Vocabulary::load('custom_vocabulary');
// Create a term and assign it to the vocabulary.
$term = Term::create([
'vid' => $vocabulary->id(),
'name' => 'New Term',
]);
$term->save();
Associating Taxonomy with Content Programmatically
1. Attaching a Term Reference Field to a Content Type:
You can programmatically attach a term reference field to a content type using the FieldConfig and FieldStorageConfig classes:
use Drupal\field\Entity\FieldConfig;
use Drupal\field\Entity\FieldStorageConfig;
// Create field storage for the term reference field.
$field_storage = FieldStorageConfig::create([
'entity_type' => 'node',
'field_name' => 'field_custom_taxonomy',
'type' => 'entity_reference',
'settings' => [
'target_type' => 'taxonomy_term',
],
]);
$field_storage->save();
// Attach the field to a content type.
$field = FieldConfig::create([
'field_storage' => $field_storage,
'bundle' => 'article', // Content type machine name.
'label' => 'Custom Taxonomy',
'description' => 'Select taxonomy terms.',
]);
$field->save();
2. Associating a Term with Content:
To associate a term with content programmatically, you'd set the term reference field's value for the specific content entity:
use Drupal\node\Entity\Node;
// Load a node (content entity).
$node = Node::load($node_id);
// Set the value of the term reference field.
$node->field_custom_taxonomy->target_id = $term_id;
$node->save();
Deleting a Vocabulary or Term Programmatically
1. Deleting a Vocabulary:
use Drupal\taxonomy\Entity\Vocabulary;
// Load and delete a vocabulary.
$vocabulary = Vocabulary::load('custom_vocabulary');
$vocabulary->delete();
2. Deleting a Term:
use Drupal\taxonomy\Entity\Term;
// Load and delete a term.
$term = Term::load($term_id);
$term->delete();
These examples demonstrate how to perform essential operations programmatically with Taxonomy in Drupal 9. Utilizing these methods, you can create, manipulate, and associate Taxonomy vocabularies and terms with content entities through code, offering flexibility and automation in managing your Drupal site's taxonomy structure.
Add new comment