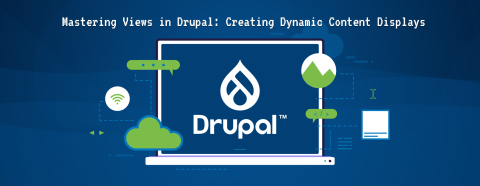
Views, an essential part of Drupal's ecosystem, allows you to create and display content in various ways. However, there are times when you need to customize Views further. This guide explores five of the most frequently used Views hooks in Drupal, providing examples and expected outcomes for each.
- hook_views_query_alter($view, $query): Tweaking the Database Query
- hook_views_pre_execute($view): Preparing for Query Execution
- hook_views_post_execute($view): Post-Query Result Manipulation
- hook_views_pre_render($view): Preparing for Rendering
- hook_views_post_render($view, $output): Post-Rendering Modifications
Let's dive into each of these hooks.
1. hook_views_query_alter($view, $query): Tweaking the Database Query
Example: Filtering Nodes with Custom Field
/**
* Implements hook_views_query_alter().
*/
function YOUR_MODULE_views_query_alter($view, $query) {
if ($view->name == 'my_custom_view') {
// Modify the query to include only nodes with a specific custom field.
$query->condition('node_field_data.field_my_custom_field_value', 'custom_value');
}
}
Expected Outcome:
In the "my_custom_view" view, the hook_views_query_alter() hook modifies the query to filter nodes with a specific custom field value ("custom_value"). As a result, only nodes with this value will be included in the view's result set.
2. hook_views_pre_execute($view): Preparing for Query Execution
Example: Adding Additional Filters Dynamically
/**
* Implements hook_views_pre_execute().
*/
function YOUR_MODULE_views_pre_execute($view) {
if ($view->name == 'dynamic_filters_view') {
// Add a dynamic filter based on user role.
$user_roles = \Drupal::currentUser()->getRoles();
if (in_array('editor', $user_roles)) {
$view->setHandler('filter', 'my_custom_filter', 'value', 'editor_value');
}
}
}
Expected Outcome:
In the "dynamic_filters_view," the hook_views_pre_execute() hook dynamically adds a filter based on the user's role. Editors will see an additional filter that allows them to filter content by "editor_value."
3. hook_views_post_execute($view): Post-Query Result Manipulation
Example: Rearranging Node Titles
/**
* Implements hook_views_post_execute().
*/
function YOUR_MODULE_views_post_execute($view) {
// Reverse the order of node titles in the view result.
$view->result = array_reverse($view->result);
}
Expected Outcome:
After executing the query, the hook_views_post_execute() hook rearranges the order of node titles in the view result. Node titles will appear in reverse order.
4. hook_views_pre_render($view): Preparing for Rendering
Example: Adding a Class to Teaser View Mode
/**
* Implements hook_views_pre_render().
*/
function YOUR_MODULE_views_pre_render($view) {
if ($view->name == 'teaser_view') {
// Add a CSS class to all teasers.
foreach ($view->result as $row) {
$row->_field_data['nid']['entity']->content['#attributes']['class'][] = 'teaser';
}
}
}
Expected Outcome:
In the "teaser_view," the hook_views_pre_render() hook adds a CSS class, "teaser," to all teasers. This class will be applied to the rendered HTML of each teaser.
5. hook_views_post_render($view, $output): Post-Rendering Modifications
Example: Replacing Text in Rendered Output
/**
* Implements hook_views_post_render().
*/
function YOUR_MODULE_views_post_render($view, &$output) {
// Replace "Old Text" with "New Text" in the view output.
$output = str_replace('Old Text', 'New Text', $output);
}
Expected Outcome:
After rendering the view, the hook_views_post_render() hook replaces all instances of "Old Text" with "New Text" in the rendered output.
Conclusion:
These commonly used Views hooks offer a wide range of possibilities for customizing and extending your Drupal site's content displays. By mastering these hooks and understanding their real-world applications, you can craft dynamic and highly customized views that precisely match your site's design and functionality requirements.
Further Reading:
With these commonly used Views hooks in your toolkit, you have the power to create dynamic, customized, and highly functional views in Drupal. These hooks offer a wealth of opportunities for fine-tuning the way your content is queried, manipulated, and presented to your audience. By understanding and effectively implementing these hooks, you can elevate the user experience and tailor content displays to meet your specific site requirements.
Add new comment