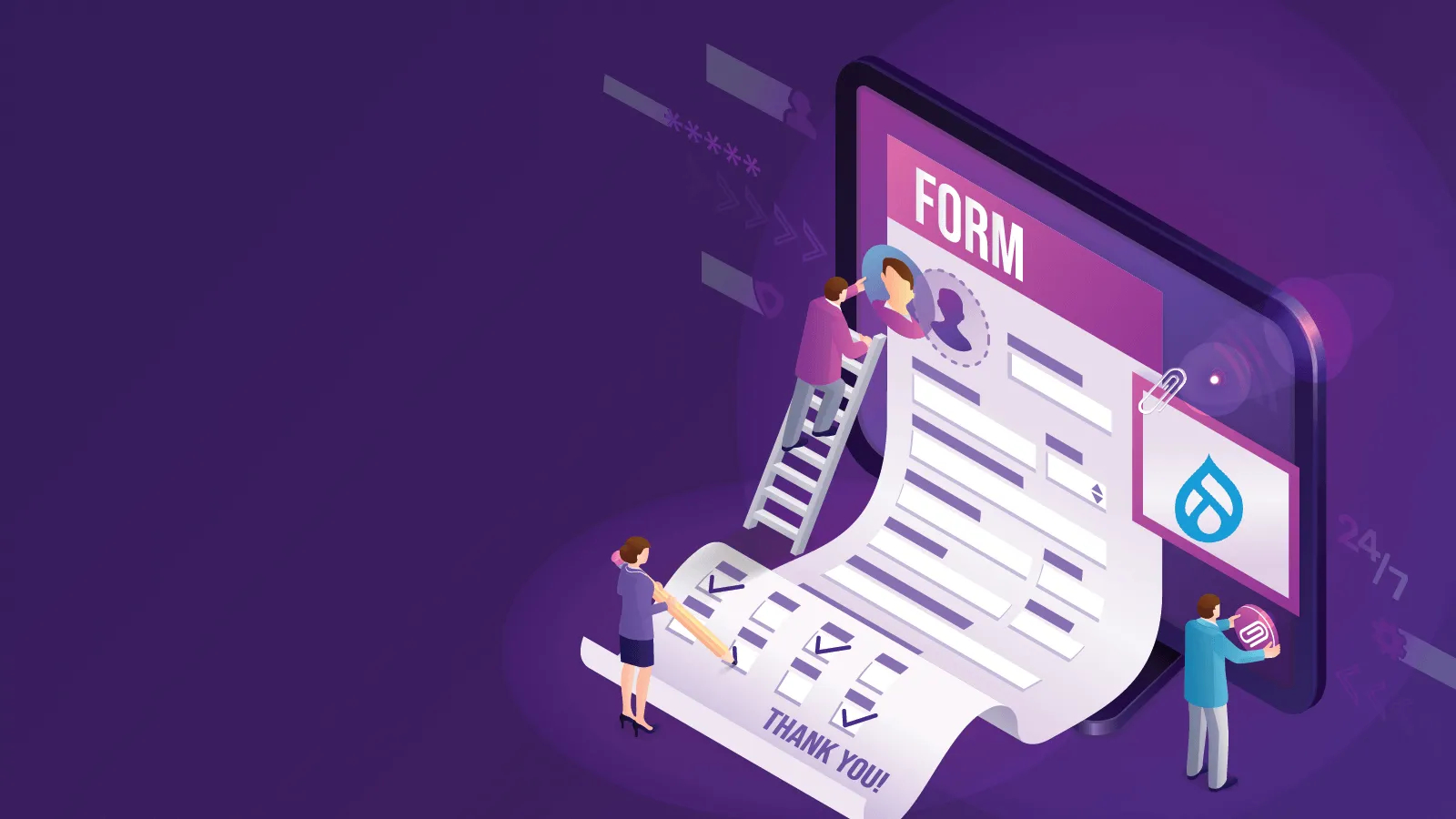
In the realm of Drupal 9, creating custom forms is a common task. To build robust and interactive forms, you need to understand and harness the power of FormState. In this blog post, we'll dive into the world of FormState and provide a comprehensive guide on how to use it in your custom forms.
What is FormState in Drupal 9?
FormState is an integral part of the Drupal Form API, responsible for managing and tracking the state of a form during its lifecycle. It allows you to access and modify user input, apply validation, and handle form submissions. FormState ensures that data remains persistent between page requests while keeping track of validation errors and user interactions.
Creating a Custom Form:
Before we delve into using FormState, let's create a simple custom form in Drupal 9. Here's an example of how to define a custom form.
use Drupal\Core\Form\FormBase;
use Drupal\Core\Form\FormStateInterface;
class MyCustomForm extends FormBase {
public function getFormId() {
return 'my_custom_form';
}
public function buildForm(array $form, FormStateInterface $form_state) {
$form['name'] = [
'#type' => 'textfield',
'#title' => $this->t('Name'),
];
$form['submit'] = [
'#type' => 'submit',
'#value' => $this->t('Submit'),
];
return $form;
}
public function submitForm(array &$form, FormStateInterface $form_state) {
// Handle form submission.
}
}
Using FormState:
Now, let's explore how to use FormState within our custom form.
1. Accessing User Input:
To access user input submitted through the form, you can use the getValue method of FormState.
public function submitForm(array &$form, FormStateInterface $form_state) {
$name = $form_state->getValue('name');
// Process and store user input.
}
2. Applying Validation:
FormState allows you to apply custom validation to form elements. You can use the setError method to set validation errors if user input doesn't meet your criteria.
public function validateForm(array &$form, FormStateInterface $form_state) {
$name = $form_state->getValue('name');
if (strlen($name) < 3) {
$form_state->setErrorByName('name', $this->t('Name must be at least 3 characters.'));
}
}
3. Redirect After Submission:
You can use FormState to redirect the user to a specific page after the form is submitted.
public function submitForm(array &$form, FormStateInterface $form_state) {
// Process form submission.
$form_state->setRedirect('your.redirect.route');
}
4. Storing Temporary Data:
FormState allows you to store temporary data that persists across page requests. This can be useful for multi-step forms.
public function buildForm(array $form, FormStateInterface $form_state) {
// Store temporary data in FormState.
$form_state->set('step', 1);
// ...
}
public function submitForm(array &$form, FormStateInterface $form_state) {
// Retrieve temporary data from FormState.
$step = $form_state->get('step');
// ...
}
Conclusion:
FormState is a powerful tool in Drupal 9 that enables you to manage user input, apply validation, and process form submissions. It plays a vital role in creating interactive and user-friendly custom forms. Whether you need to access submitted data, perform validation, or manage the flow of your form, FormState is your go-to tool.
By understanding how to use FormState effectively, you can create custom forms that meet your specific requirements, ensuring a seamless and engaging user experience on your Drupal website.
Further Reading:
With this knowledge, you're well-equipped to harness the power of FormState in your Drupal 9 custom forms, making your forms interactive and user-friendly.
Add new comment