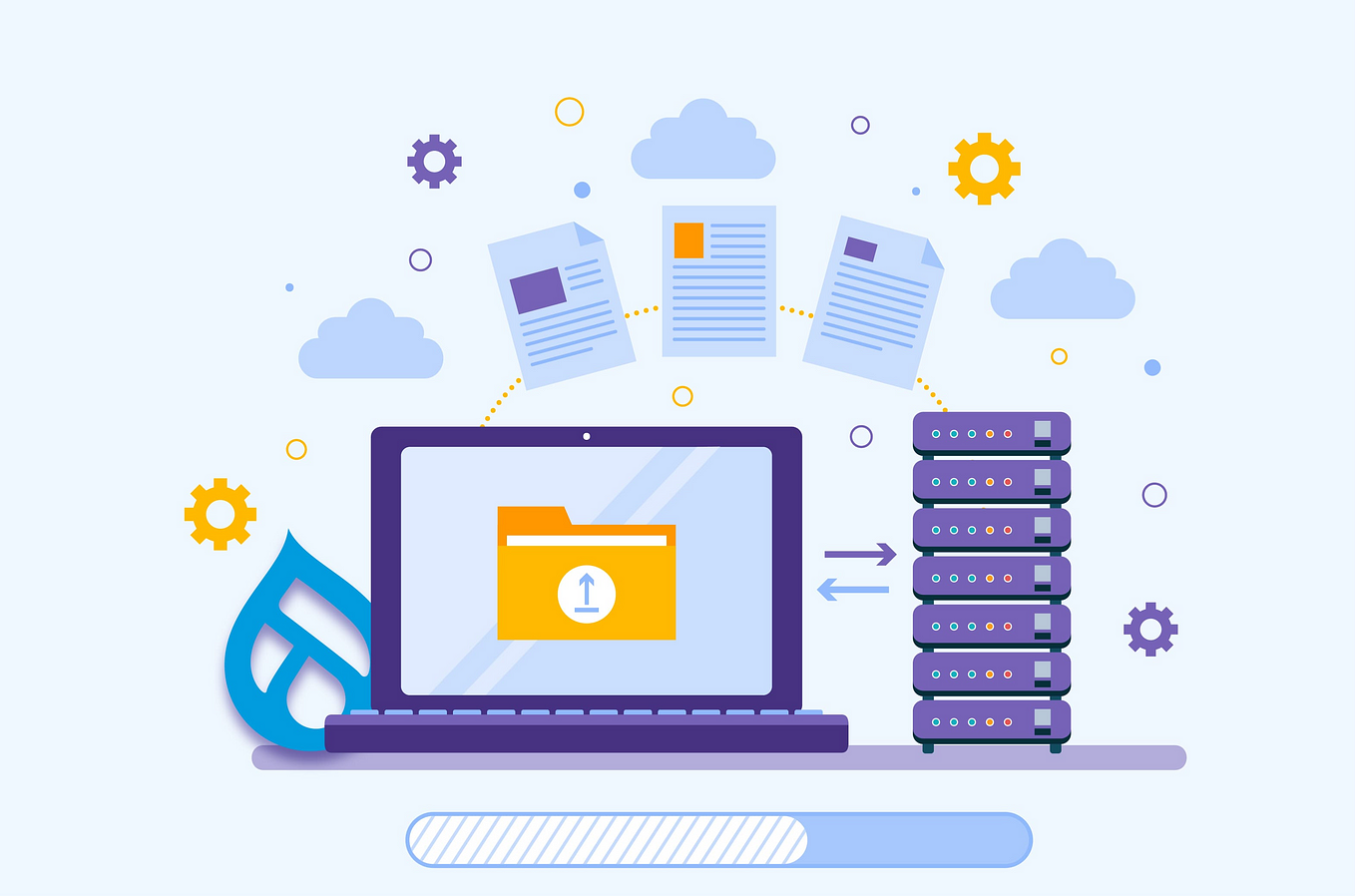
Forms are the lifeblood of interaction on the web. They enable users to input data, perform searches, submit feedback, and more. In Drupal 10, the Form API stands as a pillar of strength, empowering developers and site builders to craft forms that align perfectly with their project's requirements.
The Crucial Role of Form API in Drupal 10
In the realm of Drupal 10, the Form API serves as a powerful bridge between the user interface and the backend of a website. It dictates how forms are constructed, displayed, validated, and processed. In essence, the Form API governs the entire lifecycle of a form.
Understanding how Drupal forms are structured is fundamental to wielding the Form API effectively. In Drupal, forms are essentially arrays. Every form element, be it a simple textfield or a complex group of checkboxes, is represented as an item within this structured array.
This structured approach not only simplifies the creation and management of forms but also provides the flexibility required to craft dynamic, interactive, and customized forms.
Creating Basic Forms
Now that we've grasped the essence of the Drupal 10 Form API, it's time to dive into practicality by creating our very first form.
Building Your Inaugural Drupal 10 Form
The journey begins by defining a form function. This function serves as the entry point for your form and contains all the form-building logic. Inside this function, we employ the Form API to add form elements. These elements can range from basic textfields, radios, and checkboxes to more complex components.
Adding Form Elements: More than Meets the Eye
In Drupal, form elements are defined as array items within the form array. Each element is characterized by an array that includes properties such as the element type, title, and default value.
For instance, to include a textfield for a user's name, you'd define it like this:
$form['name'] = [
'#type' => 'textfield',
'#title' => t('Name'),
'#default_value' => '',
];
By defining these elements, you're not only creating the visual representation of the form but also determining how the data will be processed when the user interacts with it.Creating basic forms in Drupal 10 is an essential skill that serves as the foundation for more advanced form development.
Enforcing Data Integrity: Form Validation
Form validation is the shield that safeguards your web application from erroneous or malicious user input. It ensures that the data submitted aligns with the expected format and criteria. Drupal 10 simplifies this process through its built-in validation functions and methods.
You can assign validation rules to form elements by defining the #element_validate
property. For example, to validate that a phone number contains only numeric characters, you can use the number_field_validate
function.
$form['phone'] = [
'#type' => 'tel',
'#title' => t('Phone Number'),
'#default_value' => '',
'#element_validate' => ['number_field_validate'],
];
Handling the Outcome: Form Submission
Once the data is validated, the journey continues with form submission. Drupal provides a dedicated function, known as the form submission handler. By specifying the submission handler for a form element using the #submit
property, you determine how the form data will be processed, stored, or utilized within your application.
$form['submit'] = [
'#type' => 'submit',
'#value' => t('Submit'),
'#submit' => ['my_custom_form_submit'],
];
In the my_custom_form_submit function, you have the power to define the logic for processing user-submitted data, storing it in a database, or executing any other custom actions needed for your application.Form validation and submission are pivotal aspects of creating functional and secure forms in Drupal 10. As we progress, we'll delve into the world of crafting custom form elements tailored to your project's specific needs.
Crafting Your Custom Form Elements
Custom form elements offer the versatility needed to capture specific types of data, implement intricate user interactions, or seamlessly integrate third-party libraries into your forms.
In Drupal, you can create your own form element type, define its behavior, and control how it's rendered on the page. This level of customization is invaluable when you need to gather data that doesn't fit within the constraints of standard form elements.
$form['my_custom_element'] = [
'#type' => 'my_custom_element_type',
'#title' => t('Custom Element'),
// Additional properties and settings.
];
Creating custom form elements in Drupal 10 is having a toolkit filled with unique tools, each designed for a specific task. This capability opens doors to creating forms that seamlessly align with your project's requirements.
Dynamic Forms with Ajax
The world of web applications has evolved to include dynamic and interactive interfaces that respond to user actions in real time. Drupal 10's Form API allows you to create such dynamic forms through the power of Ajax.
Unveiling Ajax in Drupal Forms
Ajax in Drupal enables the creation of responsive forms that update content on the page based on user input without requiring a full page reload. This capability opens the door to various possibilities, including auto-updating dropdowns, conditional visibility of form elements, and dynamically loading additional form fields.
By defining Ajax behaviors for specific form elements, you dictate how data is fetched from or submitted to the server, all while keeping the user's experience smooth and interruption-free.
$form['dynamic_element'] = [
'#type' => 'textfield',
'#title' => t('Dynamic Element'),
'#default_value' => '',
'#ajax' => [
'callback' => 'my_ajax_callback_function',
'event' => 'change',
'wrapper' => 'dynamic-element-wrapper',
],
];
Form Theming and Styling
Drupal empowers you to override the default markup and styling of form elements using theming functions. By crafting custom theme templates, you take control over how form elements are rendered within your Drupal theme
function mytheme_theme() {
return [
'custom_form_element' => [
'render element' => 'element',
],
];
}
Advanced Form API Techniques
Multi-Step Forms
Multi-step forms, often referred to as "wizard" forms, allow you to break a lengthy form into manageable sections. This not only improves user experience but also provides opportunities to gather information progressively.
You can implement multi-step forms in Drupal by leveraging the Form API's capabilities, including the #step
property and the use of session storage for maintaining state between steps.
Form Alter Functions
Form alter functions are powerful tools for modifying existing forms created by other modules or core. You can use form alter functions to add, remove, or alter form elements and their behaviors.
By understanding the Form API's form alter hooks, such as hook_form_alter
and hook_form_FORM_ID_alter
, you can customize the behavior of forms throughout your Drupal application.
Real-World Examples and Use Cases
Contact Forms
Contact forms are a fundamental part of many websites, enabling users to get in touch with site administrators, support teams, or other users. We'll explore how to create customizable contact forms that suit your website's needs.
User Registration Forms
User registration forms are essential for building online communities and memberships. We'll guide you through creating registration forms with custom fields and validation rules to streamline user onboarding.
Content Submission Forms
Content submission forms empower your website's users to contribute articles, blog posts, or other content types. We'll cover how to design and implement submission forms that maintain data integrity and consistency.
Form Security and Best Practices
Security is a paramount concern when dealing with forms in web applications. In this chapter, we'll delve into the best practices for securing your Drupal 10 forms against potential threats and vulnerabilities.
Cross-Site Request Forgery (CSRF) Protection
CSRF attacks can pose a significant risk to your web application's security. We'll discuss how to implement CSRF protection in Drupal forms to safeguard against these types of attacks.
Input Validation and Sanitization
Ensuring that user input is valid and sanitized is crucial for maintaining data integrity and preventing security breaches. We'll explore best practices for validating and sanitizing form data to protect your application.
Optimizing Form Performance
Caching Techniques
Caching can significantly enhance form load times and reduce server load. We'll cover caching strategies for forms, including client-side caching and server-side caching techniques.
Minimizing Form Rendering Times
Efficient rendering of forms is critical for a smooth user experience. We'll discuss techniques for minimizing form rendering times by optimizing your code and utilizing Drupal's caching mechanisms.
Conclusion
Throughout this comprehensive guide, we've embarked on a journey through the Drupal 10 Form API, from understanding its basics to mastering advanced techniques. By following the principles and practices outlined, you're well-equipped to create dynamic, customized, secure, and high-performance forms that enhance user experiences on your Drupal website.
Add new comment