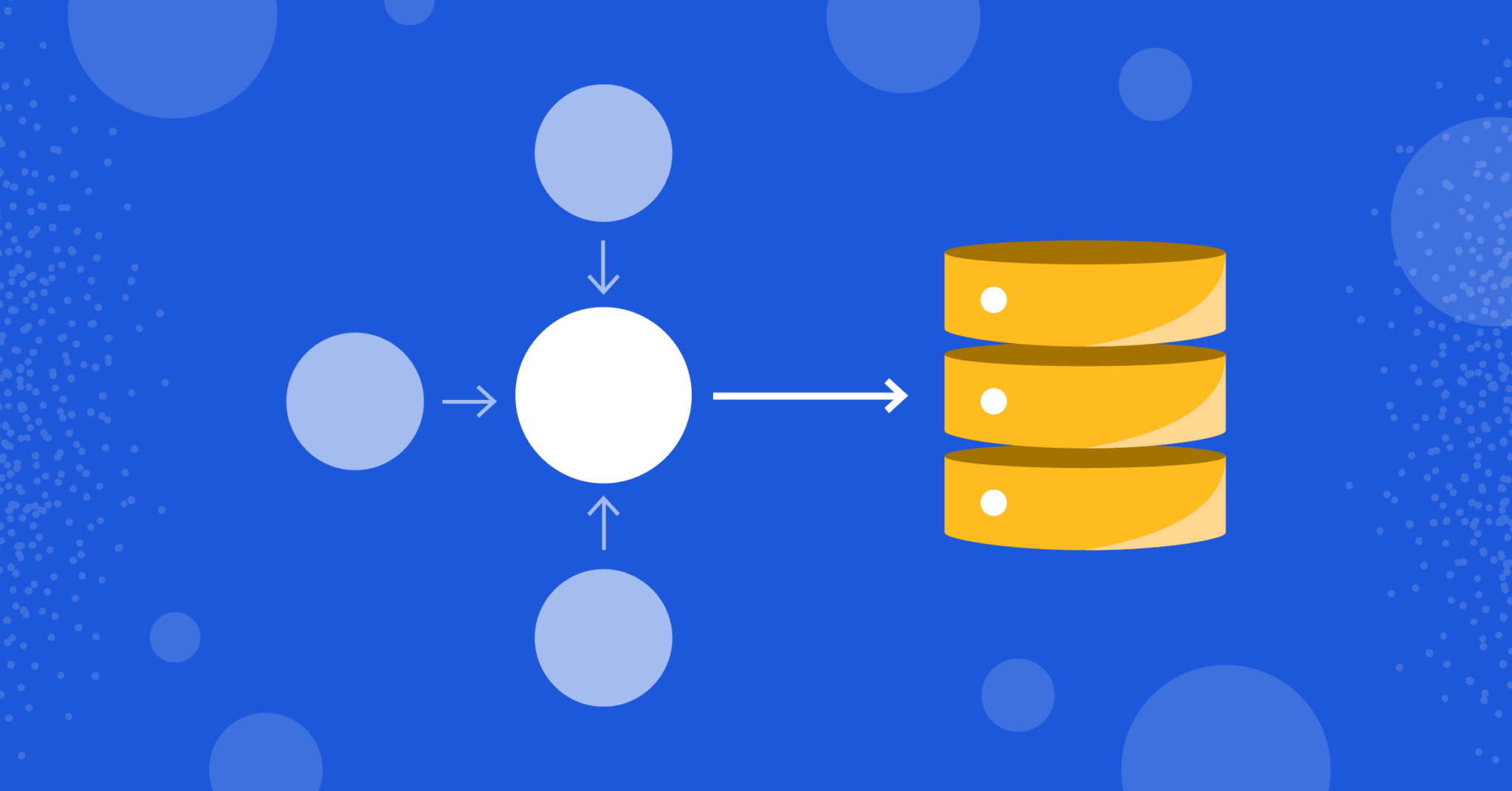
Drupal, as a robust content management system, relies heavily on its database layer for data storage and retrieval. Understanding the database classes in Drupal can empower developers to interact with the database efficiently. Let's explore the key database classes and their functionalities within Drupal.
1. DatabaseConnection Class
The DatabaseConnection class serves as the primary interface for interacting with the database. It provides methods for executing queries, managing transactions, and handling database connections.
Example Usage:
use Drupal\Core\Database\Database;
// Get the default database connection.
$connection = Database::getConnection();
// Execute a query.
$result = $connection->query('SELECT * FROM {users}')->fetchAll();
2. QueryInterface and Query Class
The QueryInterface and Query classes facilitate the construction and execution of database queries in a structured manner. They allow developers to create database-agnostic queries, enhancing portability across different database engines supported by Drupal.
Example Usage:
use Drupal\Core\Database\Connection;
use Drupal\Core\Database\Query\Select;
// Create a SELECT query.
$query = \Drupal::database()->select('node', 'n');
$query->fields('n', ['nid', 'title']);
$query->condition('n.type', 'article');
$result = $query->execute()->fetchAll();
3. EntityQuery Class
The EntityQuery class is specifically designed for querying entities within Drupal. It simplifies entity-specific queries and provides an object-oriented way to retrieve entity data.
Example Usage:
use Drupal\Core\Entity\Query\QueryFactory;
// Get the entity query service.
$queryFactory = \Drupal::service('entity.query');
$query = $queryFactory->get('node');
$query->condition('type', 'article');
$result = $query->execute();
4. Schema Classes
Drupal provides schema classes (Schema and SchemaInterface) that assist in managing database schemas, defining tables, fields, and keys within the database.
Example Usage:
use Drupal\Core\Database\Connection;
use Drupal\Core\Database\Schema\Schema;
// Get the database connection and schema service.
$connection = Database::getConnection();
$schema = new Schema($connection);
// Define a custom table schema.
$tableSchema = [
'description' => 'Custom table for storing data.',
'fields' => [
'id' => [
'type' => 'serial',
'unsigned' => TRUE,
'not null' => TRUE,
],
'name' => [
'type' => 'varchar',
'length' => 255,
'not null' => TRUE,
],
],
'primary key' => ['id'],
];
$schema->createTable('custom_table', $tableSchema);
Understanding and utilizing these database classes in Drupal empowers developers to interact with the database layer effectively, execute queries efficiently, manage database schemas, and retrieve entity-specific data. This knowledge enables the development of robust and efficient Drupal applications while ensuring proper database interactions and query optimizations.
Add new comment