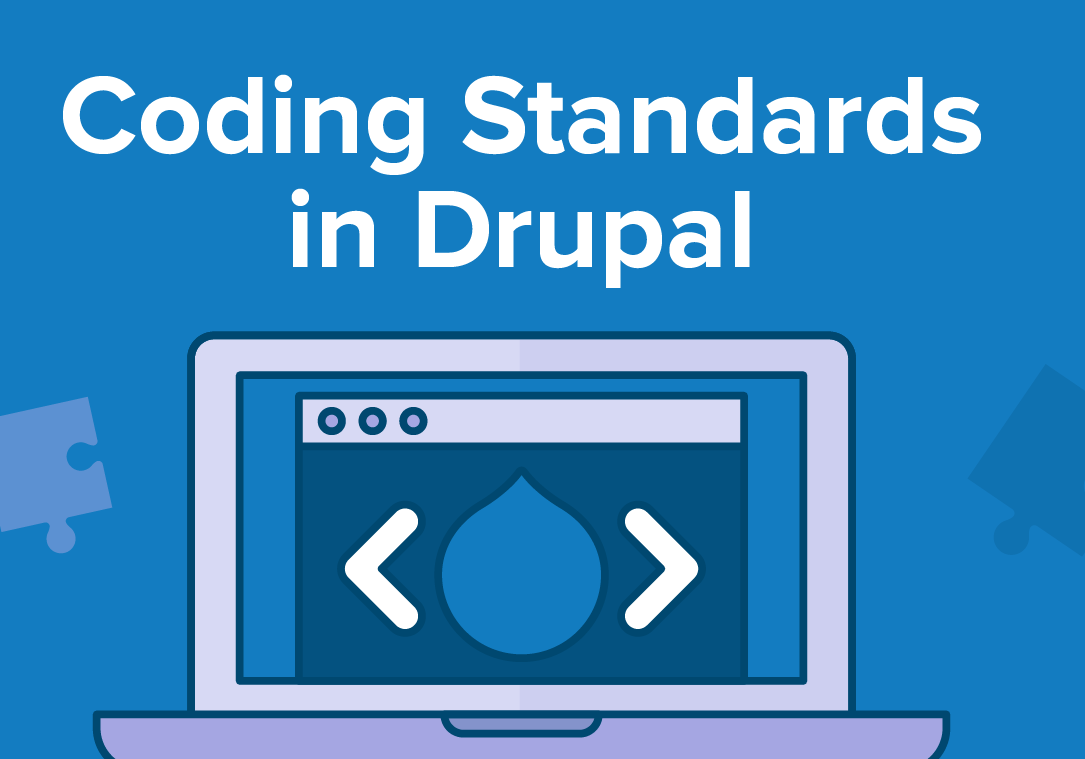
Consistency, readability, and maintainability are paramount in Drupal 9 projects. Coding standards serve as guiding principles to achieve these goals. Let's explore practical code examples that demonstrate the implementation of coding standards within the Drupal 9 ecosystem.
Consistency for Clarity and Cohesion
Example: PSR-1 and PSR-2 Standards
Following PSR-1 and PSR-2 standards ensures code consistency. For instance, adhering to these standards in class and method naming:
// PSR-1: Class names should be declared in StudlyCaps.
class ExampleClass {
// PSR-1: Method names should be declared in camelCase.
public function exampleMethod() {
// PSR-2: Use 4 spaces for indentation.
$variable = 'Example';
if ($condition) {
// PSR-2: Use spaces for indentation, not tabs.
// PSR-2: Use single quotes for string literals.
$variable = 'Another Example';
}
}
}
Readability for Seamless Collaboration
Example: Drupal Naming Conventions
Adhering to Drupal's naming conventions ensures readability and ease of collaboration among developers:
// Drupal: Function names should use underscores for spaces.
function example_function_name() {
// Drupal: Variables should also use underscores.
$example_variable = 'Example';
}
Sustaining Maintainability and Longevity
Example: Documentation Blocks
Maintaining well-documented code enhances maintainability. Following Drupal's standards for documentation blocks
/**
* Implements hook_menu().
*
* Adds a menu item for the custom module.
*/
function custom_module_menu() {
// Function implementation.
}
Implementing Coding Standards in Drupal 9 Tooling
Example: Code Sniffer Integration
Using PHP Code Sniffer with Drupal coding standards for validating code:
# Run PHP Code Sniffer with Drupal coding standards.
$ phpcs --standard=Drupal path/to/my/module
Example: IDE Integration for Validation
Integrating coding standards checks within PhpStorm using relevant plugins:
https://www.jetbrains.com/help/phpstorm/drupal-support.html
Example: CI/CD Configuration
Configuring CI/CD tools like Travis CI to perform automated coding standard checks:
# .travis.yml file configuration
script:
- phpcs --standard=Drupal path/to/my/module
Conclusion: Elevating Code Quality in Drupal 9
Implementing coding standards in Drupal 9 is essential for fostering maintainable, collaborative, and high-quality projects. Consistency in adhering to PSR and Drupal standards, readability through clear naming conventions, and tooling integration for automated checks are pivotal for code excellence.
By embracing coding standards, Drupal 9 developers contribute to a culture of excellence, ensuring robust, sustainable, and long-lasting projects within the Drupal ecosystem.
Add new comment